Using animejs for animations"
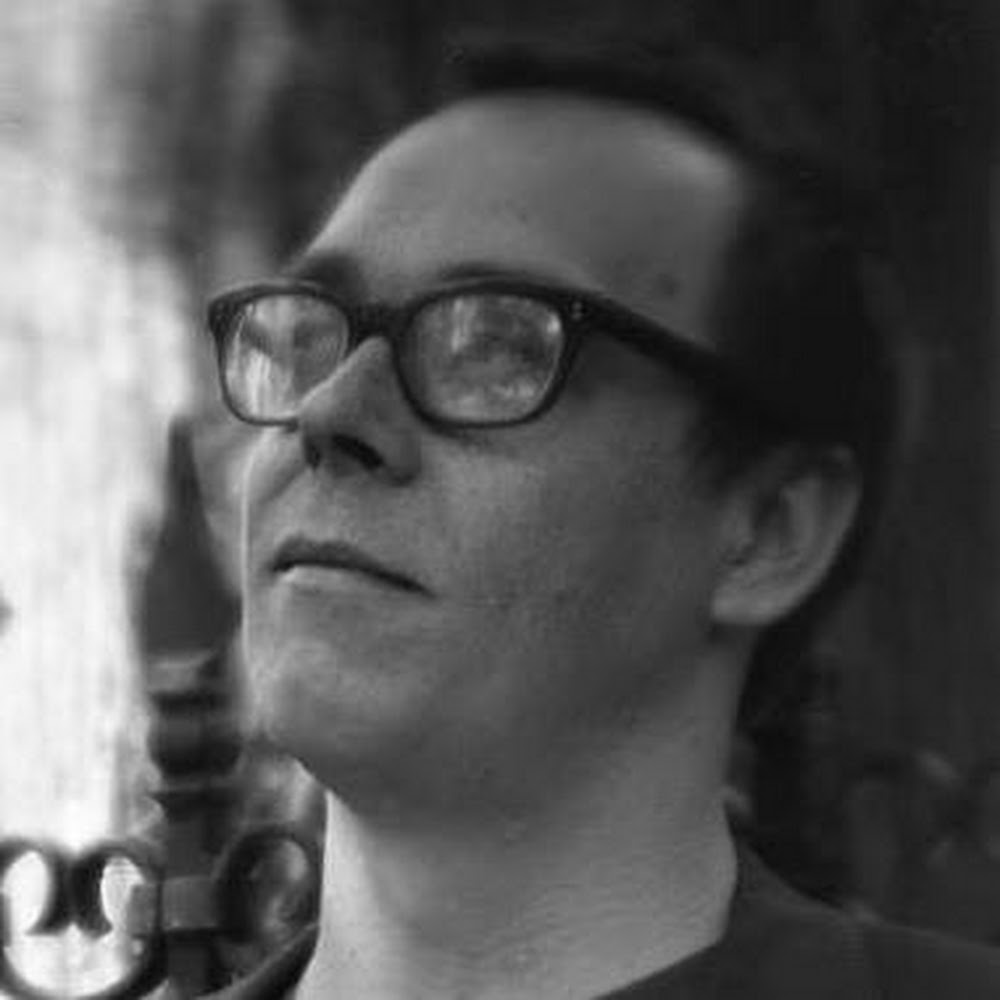
Human
You're an AI master at writing javascript code, you are given a new library name, a short description and a set of urls describing it. Please read the information online describing how the tool should be used and then provide a non-trivial real world to-do list example of it. Library: animejs URLs: https://github.com/juliangarnier/anime https://animejs.com/documentation
gpt-4
Anime.js is a lightweight JavaScript animation library with a simple, yet powerful API. It works with any CSS properties, individual CSS transforms, SVG, or any DOM attributes, and JavaScript Objects. You can find more information about the library on its GitHub page (https://github.com/juliangarnier/anime) and the official documentation (https://animejs.com/documentation).
Based on the information provided, let's create a non-trivial real-world to-do list example using Anime.js.
- First, include the Anime.js library in your HTML file:
html
Copy
<script src="https://cdnjs.cloudflare.com/ajax/libs/animejs/3.2.1/anime.min.js"></script>
- Create the HTML structure for the to-do list:
html
Copy
<div id="todo-list">
<input type="text" id="todo-input" placeholder="Add a task">
<button id="add-task">Add Task</button>
<ul id="task-list"></ul>
</div>
- Add some basic CSS styles:
css
Copy
#todo-list {
width: 300px;
margin: auto;
}
#task-list {
list-style-type: none;
padding: 0;
}
.task-item {
background-color: #f0f0f0;
padding: 10px;
margin-bottom: 5px;
cursor: pointer;
}
- Now, let's write the JavaScript code to handle adding tasks and animating them using Anime.js:
javascript
Copy
document.getElementById('add-task').addEventListener('click', addTask);
function addTask() {
const taskInput = document.getElementById('todo-input');
const taskList = document.getElementById('task-list');
const taskText = taskInput.value.trim();
if (taskText) {
const taskItem = document.createElement('li');
taskItem.classList.add('task-item');
taskItem.textContent = taskText;
taskItem.addEventListener('click', () => {
anime({
targets: taskItem,
opacity: [1, 0],
translateY: ['-10px', '10px'],
duration: 500,
easing: 'easeInOutQuad',
complete: () => {
taskList.removeChild(taskItem);
},
});
});
taskList.appendChild(taskItem);
anime({
targets: taskItem,
opacity: [0, 1],
translateY: ['10px', '-10px'],
duration: 500,
easing: 'easeInOutQuad',
});
taskInput.value = '';
}
In this example, we've created a simple to-do list where you can add tasks and remove them by clicking on them. When a task is added or removed, we use Anime.js to animate the task item's opacity and position.
Sources
code.tutsplus.com
www.tutorialspoint.com
Become an Animation Master with Anime.js - Setting up the Environment and Basic Animations - DEV Community
GPT-4
Share
Continue chat